Finding the Python reference docs
Since I'm a total Python beginner, I frequently need to read about its common built-in functions and idioms.
Docs
- https://docs.python.org/3/. Check out the Tutorial and Library Reference.
- Built-in functions: https://docs.python.org/3/library/functions.html
- https://docs.python.org/3/library/stdtypes.html#sequence-types-list-tuple-range
- Loops: https://docs.python.org/3/tutorial/datastructures.html#looping-techniques
- Functions: https://docs.python.org/3/tutorial/controlflow.html#defining-functions
- List comprehension - an idiomatic creation of lists: https://docs.python.org/3/tutorial/datastructures.html#list-comprehensions
- Iterating numpy ndarrays: the numpy docs mention np.nditer, but enumerate() works better.
- OS: https://docs.python.org/3/library/os.html
- File utilities: https://docs.python.org/3/library/shutil.html
- Modules and imports: https://docs.python.org/3/tutorial/modules.html
- Logical And and Logical Or: this is baffling. It is impossible to find in the official docs. See https://docs.python.org/3/library/operator.html#mapping-operators-to-functions, it shows a huge table, but no Logical And nor Logical Or. The answer is, use
and
andor
, not&&
and||
like in most other languages. - Ternary operator: https://docs.python.org/3/reference/expressions.html#conditional-expressions.
a if condition else b
. - get request: urllib is an option, but the best is
requests
.
import requests
r = requests.get('https://api.github.com/events')
print(r.json())
- pretty print json: json module.
import json
print(json.dumps({'4': 5, '6': 7}, sort_keys=True, indent=4))
- Common operations on lists:
sum(list)
,math.prod(list)
(afterimport math
). - Joining the reverse of a list into a string:
''.join(reversed(list))
Finding them the hard way
For example, I stumbled upon this snippet somewhere: max([max(sequence) for sequence in train_data])
. What does max do for a list? Not sure. So I googled "python max", expecting the first result would be the python.org documentation. Nope, it's full of third-party sites and blogs.
So I went to python.org, and typed "max" in the search box.
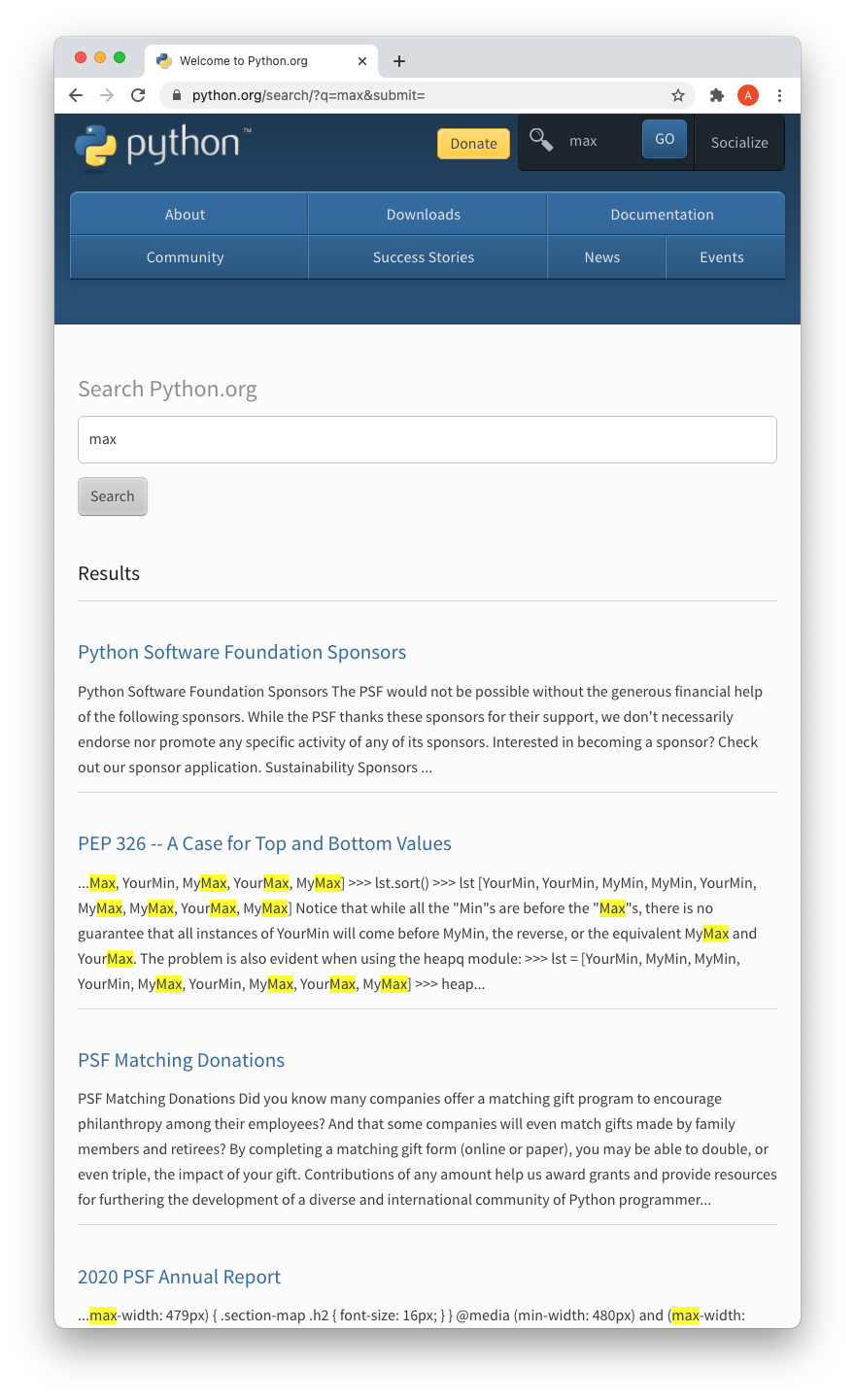
So instead of search, I looked for reference docs. But the link to the Beginner's Guide seemed broken.
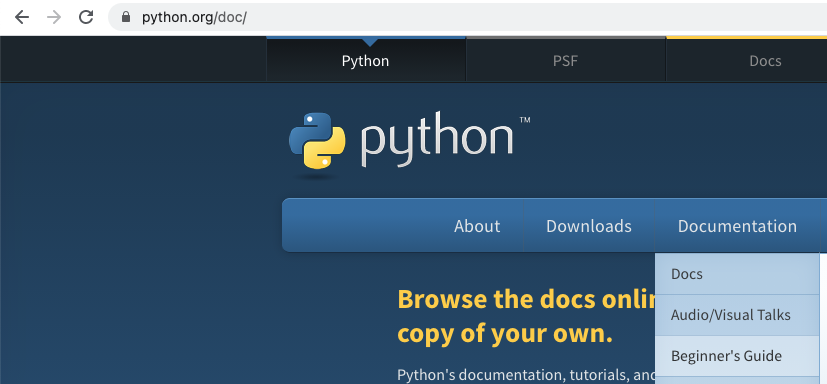
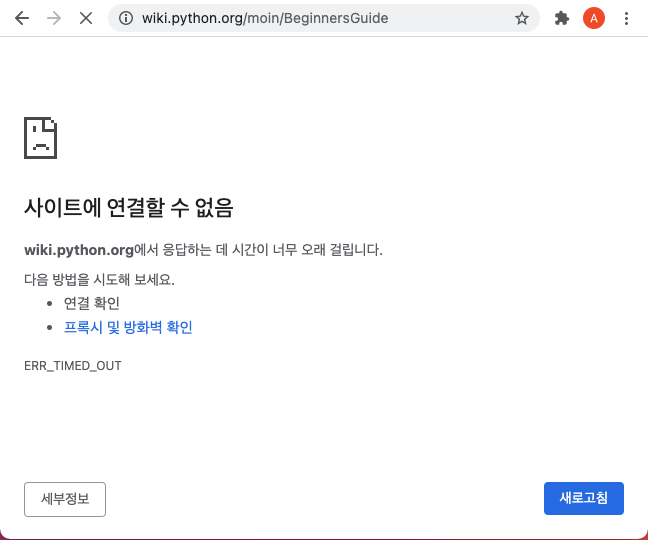
The documentation on the language also has no mention of max: https://docs.python.org/3/tutorial/datastructures.html.
It turned out, I should have looked for the docs on Python built-in library. https://docs.python.org/3/library/stdtypes.html#sequence-types-list-tuple-range.